A common Arduino system typically includes a display of some type. This project was to set up a 16×2 LCD display to an Arduino unit and run it with simple messages that render it available for inputs or computational processing. It produces an informational output of its own, or to correspond to another event controlled by a wider system.
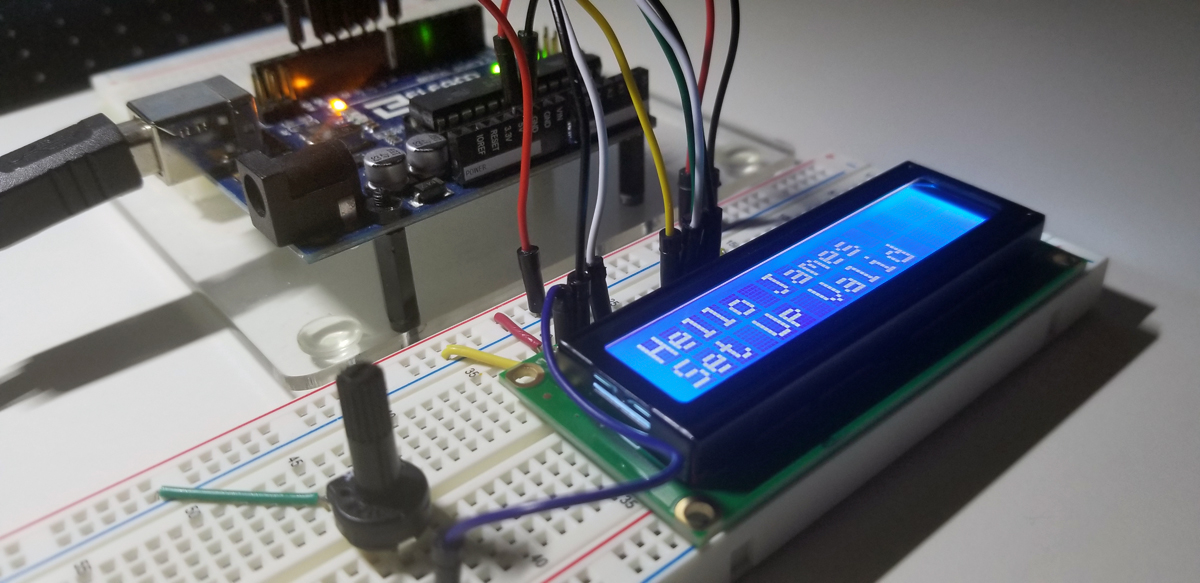
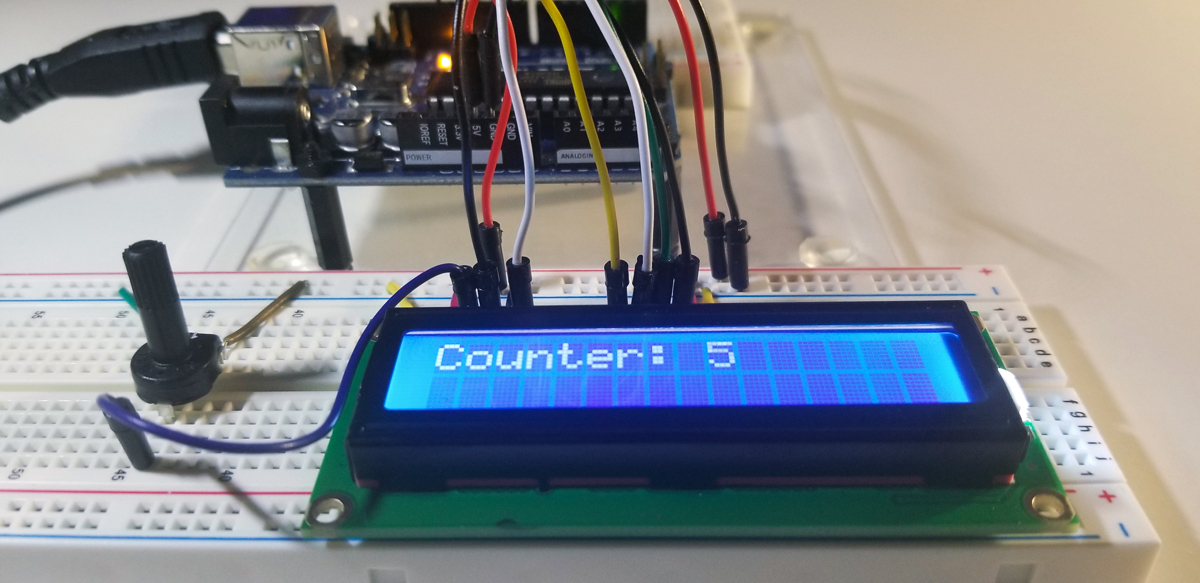
The physical connections between the Arduino and LCD display are through direct jumper connections between GPIO pins to data, control, power, and ground. The potentiometer is simply for character illumination intensity.
The digital GPIO pins that interface to the LCD connect to its data pins. R/W is strapped low to ground.
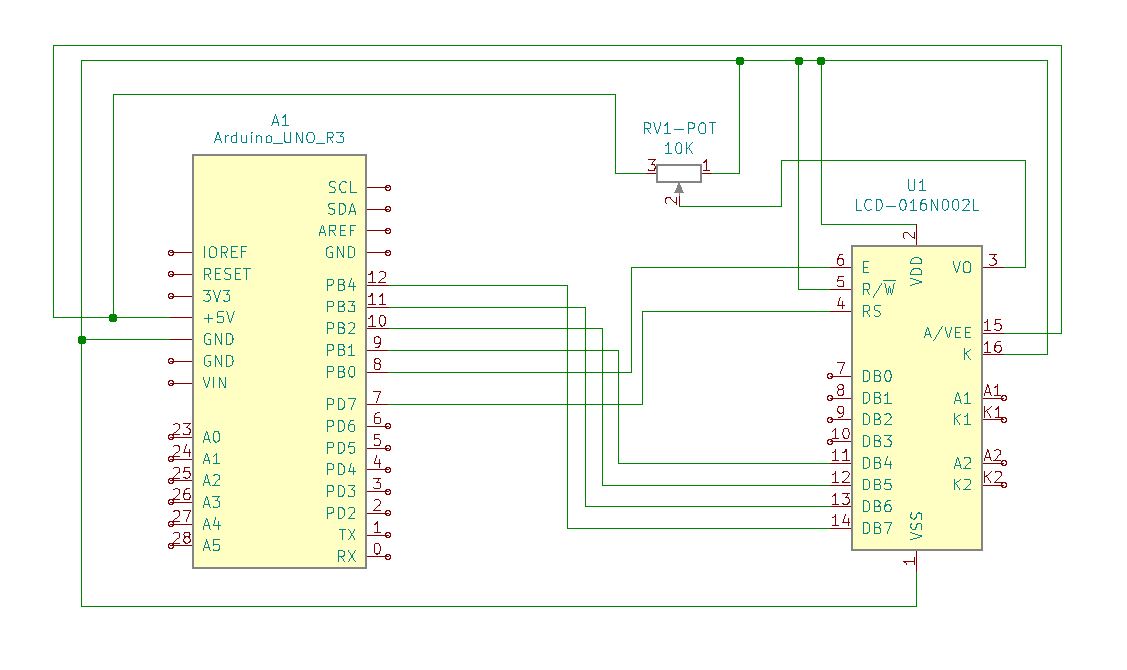
1602A LCD Display Pinout
Pin | Symbol | Function |
---|---|---|
1 | VSS | Ground |
2 | VDD | +5VDC |
3 | V0 | Contrast |
4 | RS | Register |
5 | R/W | Read/Write |
6 | E | Enable |
7 | D0 | Data |
8 | D1 | Data |
9 | D2 | Data |
10 | D3 | Data |
11 | D4 | Data |
12 | D5 | Data |
13 | D6 | Data |
14 | D7 | Data |
15 | A | Anode (+5VDC) |
16 | K | Cathode (Ground) |
Program & Functional Operation:
Code Setup:
#include <LiquidCrystal.h>
int rs=7;
int en=8;
int d4=9;
int d5=10;
int d6=11;
int d7=12;
LiquidCrystal lcd(rs,en,d4,d5,d6,d7);
void setup() {
lcd.begin(16,2);
}
void loop() {
lcd.setCursor(0,0);
lcd.print(“Counter:”);
lcd.setCursor(0,1);
lcd.print(“Hello James”);
for (int j=1;j<=10;j=j+1){
lcd.setCursor(9,0);
lcd.print(j);
delay(500);
}
lcd.clear();
}
Arduino Setup:
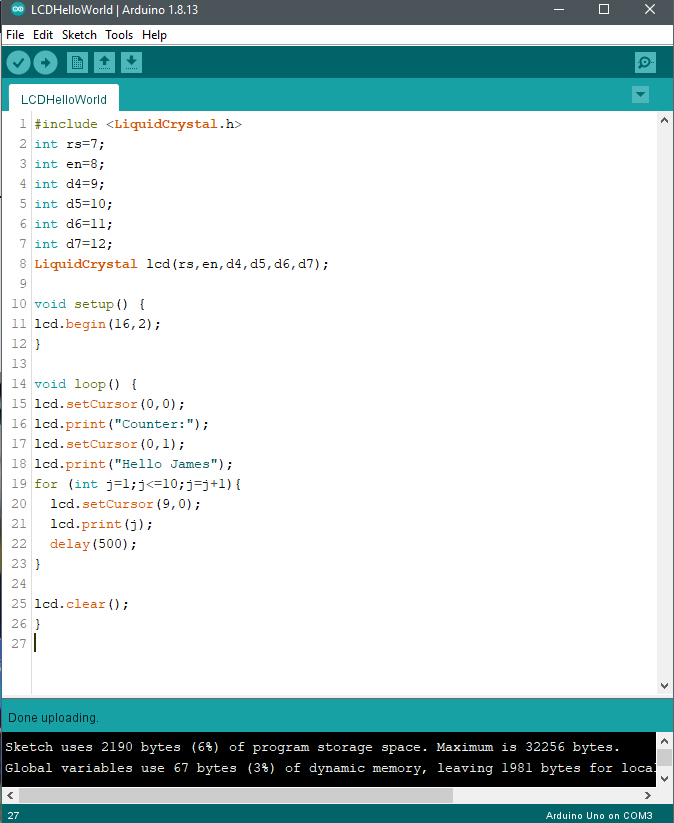
Calculator Setup:
#include <LiquidCrystal.h>
int rs=7;
int en=8;
int d4=9;
int d5=10;
int d6=11;
int d7=12;
float firstNum;
float secNum;
float answer;
String op;
LiquidCrystal lcd(rs,en,d4,d5,d6,d7);
void setup() {
lcd.begin(16,2);
Serial.begin(9600);
}
void loop() {
lcd.setCursor(0,0);
lcd.print(“Input 1st Number”);
while (Serial.available()==0){
}
firstNum=Serial.parseFloat();
lcd.clear();
lcd.setCursor(0,0);
lcd.print(“Input 2nd Number”);
while (Serial.available()==0){
}
secNum=Serial.parseFloat();
lcd.clear();
lcd.setCursor(0,0);
lcd.print(“Operator(+,-,*,/)”);
while (Serial.available()==0){
}
op=Serial.readString();
if (op==”+”){
answer=firstNum+secNum;
}
if (op==”-“){
answer=firstNum-secNum;
}
if (op==””){
answer=firstNumsecNum;
}
if (op==”/”){
answer=firstNum/secNum;
}
lcd.clear();
lcd.setCursor(0,0);
lcd.print(firstNum);
lcd.print(op);
lcd.print(secNum);
lcd.print(” = “);
lcd.print(answer);
lcd.setCursor(0,1);
lcd.print(“Thank You”);
delay(10000);
lcd.clear();
}