This project involves the use of an HC-SR04 proximity sensor commonly used among robotic devices for purposes of collision avoidance. In this project, the sensor is applied to an Arduino Nano system measure distance from an object and report back the results to a serial monitor on a computer, and an LCD display. This unit is powered via a battery or USB power back for portability.
Each measurement is taken once the sensor appears before an object, or if a target object is placed in front of the sensor. Once the target is placed, a user is prompted to press the circuit switch to activate the measurement activity that occurs. The measurement reported to a computer and LCD display is an average of 100 readings between the sensor and the placed object. The Nano programmatically operates a high-speed loop by which each reading event (0 to 100) is cumulatively stored into a memory bucket. Once all reading measurements are taken, the average measurement is obtained by calculating the total by dividing the number of measurements completed into the total bucket value. This function is repeated for each switch/button-press event to activate a measurement.

Functional Demonstration Video:
Project Schematic:
Arduino Setup:
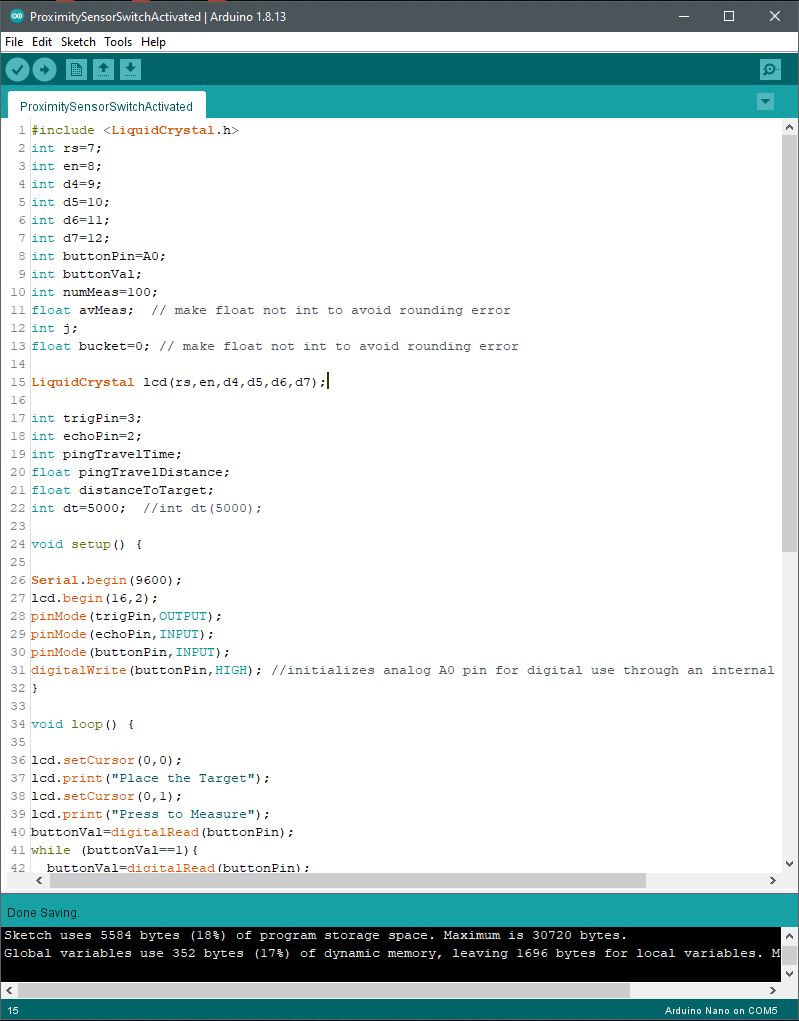
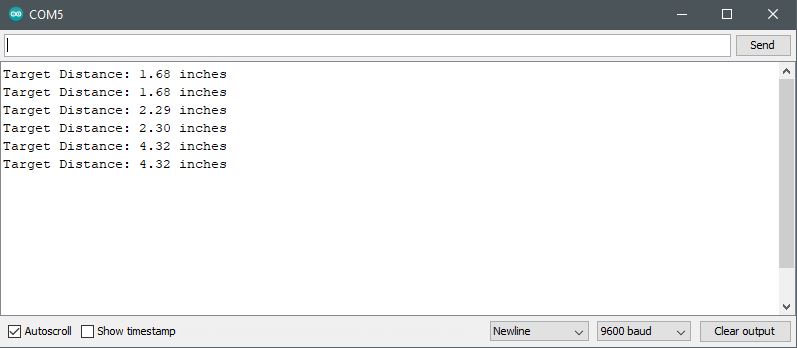
Code Example:
#include <LiquidCrystal.h>
int rs=7;
int en=8;
int d4=9;
int d5=10;
int d6=11;
int d7=12;
int buttonPin=A0;
int buttonVal;
int numMeas=100;
float avMeas; // make float not int to avoid rounding error
int j;
float bucket=0; // make float not int to avoid rounding error
LiquidCrystal lcd(rs,en,d4,d5,d6,d7);
int trigPin=3;
int echoPin=2;
int pingTravelTime;
float pingTravelDistance;
float distanceToTarget;
int dt=5000; //int dt(5000);
void setup() {
Serial.begin(9600);
lcd.begin(16,2);
pinMode(trigPin,OUTPUT);
pinMode(echoPin,INPUT);
pinMode(buttonPin,INPUT);
digitalWrite(buttonPin,HIGH); //initializes analog A0 pin for digital use through an internal pull up resistor.
}
void loop() {
lcd.setCursor(0,0);
lcd.print(“Place the Target”);
lcd.setCursor(0,1);
lcd.print(“Press to Measure”);
buttonVal=digitalRead(buttonPin);
while (buttonVal==1){
buttonVal=digitalRead(buttonPin);
}
lcd.setCursor(0,0);
lcd.clear();
lcd.print(“Measuring…”);
for (j=1;j<=numMeas;j=j+1){
digitalWrite(trigPin,LOW);
delayMicroseconds(10);
digitalWrite(trigPin,HIGH);
delayMicroseconds(10);
digitalWrite(trigPin,LOW);
pingTravelTime=pulseIn(echoPin,HIGH);
delay(25);
pingTravelDistance=(pingTravelTime*765.*5280.*12)/(3600.*1000000);
distanceToTarget=pingTravelDistance/2;
bucket=bucket+distanceToTarget;
}
avMeas=bucket/numMeas;
Serial.print(“Target Distance: “);
Serial.print(distanceToTarget);
Serial.println(” inches”);
lcd.clear();
lcd.setCursor(0,0);
lcd.print(“Target Distance:”);
lcd.setCursor(0,1);
lcd.print(distanceToTarget);
lcd.print(” Inches”);
delay(dt);
}