This project makes use of a thumb joystick to control the direction of shaft rotation on a DC motor. The joystick also controls the rotational speed of the motor shaft by how far it is moved horizontally (y-axis). With the joystick on center and at rest, the DC motor doesn’t rotate. However, gradually moving the joystick horizontally to the right increases the speed of the motor until a maximum value permitted by a L293 motor controller. The same method of variable speed control is achieved in reverse with its negative limit to available speed in the opposite rotational direction.
Further details that correspond to this photo are given in the schematic diagram below. The schematic diagram provides specific connection details to indicate where system components are placed and terminated.
Project Schematic:
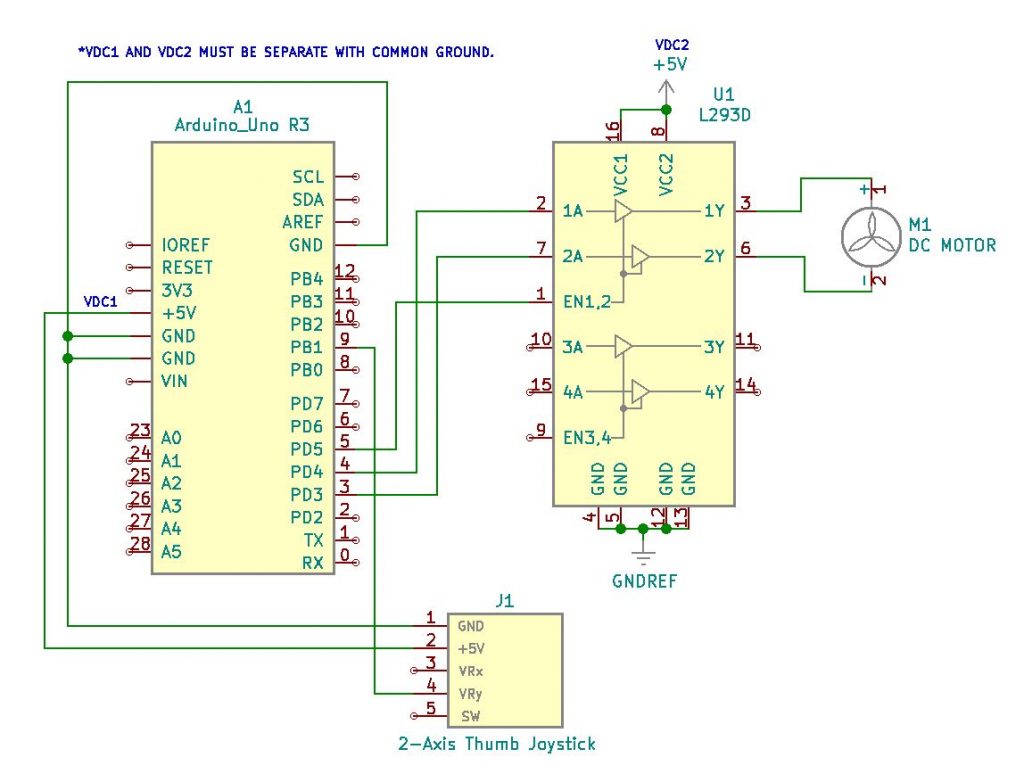
Motor Speed Formulas:
To continuously update the motor speed as a function of gradual joystick movement, it is necessary to read updated joystick values read from memory. As an assigned analog GPIO pin (A1) reads the VRy levels at the joystick, continuous updates are made to memory in order to change motor speed and direction.
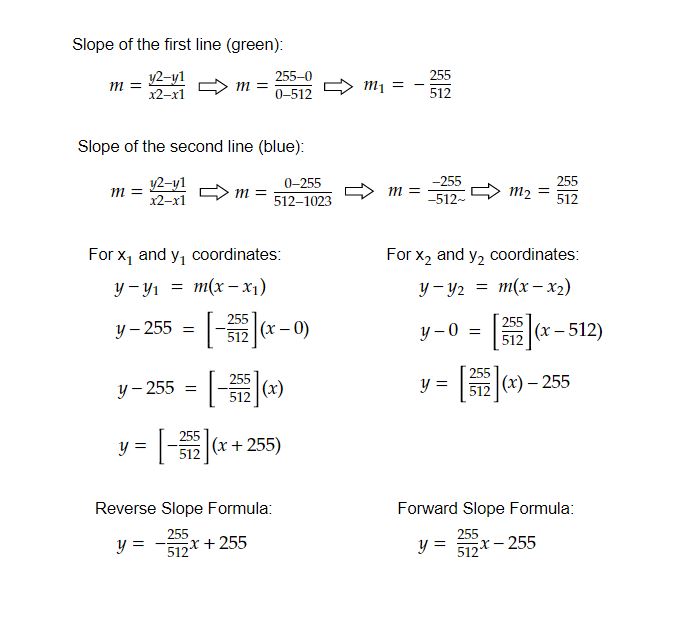
Continuously changing joystick values read into memory becomes calculated for increased or decreased speed adjustment either in a forward or reverse direction. The positive y-axis movement rotates the motor shaft clockwise while the negative y-axis joystick movement rotates the motor shaft counter-clockwise. Both at continuously variable speeds depending upon the joystick’s position off-center from its physically at-rest state.
Project Review:
It is necessary to pump the joystick to effect charge momentum for break-through motion and reversal. As demonstrated here, the positive y-axis joystick movement rotates the motor shaft clockwise while horizontally negative joystick movement rotates the shaft counterclockwise. The thumb joystick is inverted in this video, so the opposite lateral motion appears counter-intuitive. Reorient the joystick 180 degrees and its motion directly corresponds to the positive and negative movement for positive and negative rotation.
Code Example:
int speedPin=5;
int dir1=4;
int dir2=3;
int mSpeed;
int jPin=A1;
int jVal;
void setup()
{
pinMode(speedPin,OUTPUT);
pinMode(dir1,OUTPUT);
pinMode(dir2,OUTPUT);
pinMode(jPin,INPUT);
Serial.begin(9600);
}
void loop()
{
jVal=analogRead(jPin);
Serial.println(jVal);
if (jVal<505)
{
delay(25);
digitalWrite(dir1,LOW);
digitalWrite(dir2,HIGH);
mSpeed=-255./512.jVal+255.; //derived equation
delay(100);
analogWrite(speedPin,mSpeed);
}
if (jVal>=505)
{
delay(25);
digitalWrite(dir1,HIGH);
digitalWrite(dir2,LOW);
mSpeed=(255./512.)jVal-255.; //derived equation
delay(100);
analogWrite(speedPin,mSpeed);
}
}